Now that you know how to use the editor and understand Lua scripting, we're going to start pulling it all together to implement gameplay. This lesson demonstrates how to create a simple marble game using the knowledge we've learned in the previous tutorials.Because this game is pretty simple, we're going to walk through the Lua scripts in detail.
Project Setup
This lesson uses the "Marble Game" project template. To begin, select the File > Project Manager menu item in the main menu. Press the New button in the project manager window to open the New Project window.
Enter a name for your project and press the OK button to create the project. Press the OK button in the project manager window to switch to our new project and begin the tutorials.Select the File > Open menu item in the main menu and choose the "start.map" file to open. This scene just contains some ground, a directional light, and two models, a ball and a coin. The ball and coin models don't have any script attached to them, so at this point they don't do anything special. There is also no camera in the scene, so running the map at this point will just result in a black screen. This isn't much of a game at this point, so let's see what we can do to make it more fun.
Creating The Player Script
The project already contains scripts for the ball and coin behavior. However, we're going to create our own scripts from scratch so we can learn step-by-step how everything works. Use the asset browser to navigate to the "Scripts\Objects\Player" folder. Right-click anywhere in the bottom panel and select the New > Script popup menu to create a new script file. Name this file "MyBallPlayer". This is our custom player script we will work on.
Double-click on the new script to open it in the script editor. The script will already have all the available functions declared but commented out. Let's get rid of everything and start from scratch. Select the Edit > Select All menu item in the script editor and press backspace to delete all the text so we can start with a blank page.
Adding a Camera
The first thing we're going to do is add a camera that gets created in our script. We could also create a camera entity in the editor, but creating it in the script makes things easier. We'll create the camera in the Start() function so it gets created when the map is loaded. We will also add an UpdateCamera() function we can call from anywhere to adjust the camera's position so it always points at the ball. At this code to the "MyBallPlayer.lua" script:function Script:Start()
--Create a camera
self.camera = Camera:Create()
--Update the camera
self:UpdateCamera()
end--Adjust the camera orientation relative to the ball
function Script:UpdateCamera()
self.camera:SetRotation(45,0,0)
self.camera:SetPosition(self.entity:GetPosition())
self.camera:Move(0,0,-4)
end
If you're ever not sure what a command does, check the API Reference for a description and examples.
At this point, the script isn't attached to an entity, so if we run the game it won't get used. We're going to attach this script to our ball model. Select the ball model by left-clicking on it in the 3D viewport. Select the Scene tab in the side panel to view the scene panel. The object's properties will be shown in the lower half of the scene panel:
This model already has a couple of properties set up to get it ready for the game. Under the appearance tab, the diffuse color has been set to blue to make it more interesting. You can change this color to your own if you wish. More importantly, under the physics tab, the mass has already been set to 1.0. By default, objects have zero mass and will not react to physics forces. By setting the mass to a value greater than zero, we allow the entity to react to physics forces, which we will use to move the ball.Now we're going to add our new script to the ball model so that our code gets executed. Select the Script tab in the properties editor. Next to the Script property, press the button marked with a folder. This will open a file selection dialog where you can choose the script file "Scripts\Objects\Player\MyBallPlayer.lua:
Press the Open button, and the script property will now be set to our custom player script:
Press F5 to run the game, and we will now be able to see the scene from the point of view of the camera.
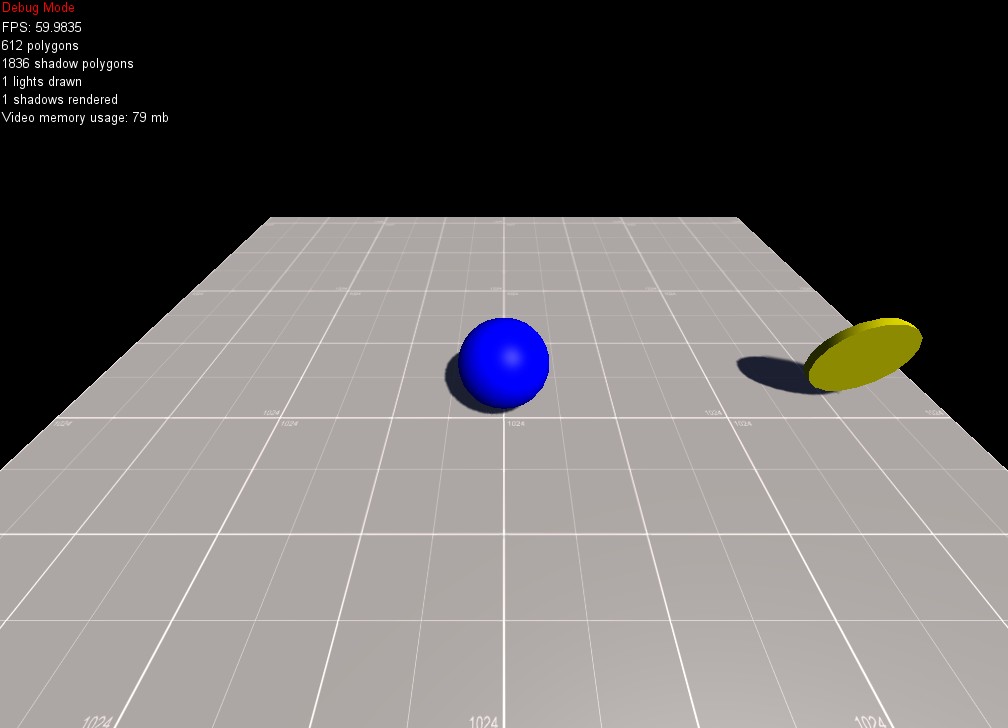