Powerups are a great way to motivate the player to go where you want and reward them for accomplishing a difficult task. Think about some of the games you have played and the different types of powerups they may have used. This includes weapons, ammo, special items in your inventory, as well as health packs and even money. In our game we're going to use the coin to reward the player, and give them a goal of collecting all the coins they can find.You might even have tried to pick up the coin when we added player controls. If you did, you would see that the player simply goes right through the coin:
Let's take a closer look at the coin model's properties. Select this object and take a look at the physics tab in the properties editor. Notice that the collision type if set to "Trigger". This is important because we want to be able to detect collision between the player and the coin, but we don't want to player to bounce off the coin when they hit. So right now although the collision between to two is being detected, there's no script that takes any action on that event, so it looks like nothing is happening.
We're going to create a custom script for the coin, in the same way we did for the player. In the asset browser, navigate to the "Scripts\Objects\Coin" folder. Create a new script here and name it "MyCoin.lua".
Switch back to the scene panel and select the Scripts tab in the properties editor. Press the button marked with a folder icon and choose the script we just created, "Scripts\Objects\Coin\MyCoin.lua".
Press the Open button in the file open dialog and our new script will now be attached to the coin model.
At this point, our coin script still doesn't do anything, so let's open it in the script editor. Select the Edit > Select All menu item in the script editor menu to select all text, then press backspace to delete it all. Now let's add some simple code that makes the coin spin around slowly in place. This will make it more apparent that the coin is an object we can interact with. Paste this code into the script editor, run the game, and you will see the coin slowly spinning in place:
function Script:UpdateWorld()
self.entity:Turn(0,Time:GetSpeed(),0,true)
end
Now we will add some code to make our coin react when the player touches it.When the player picks up the coin, we want a few different things to happen. First, we want a sound to play. This helps communicate to the player that something good happened, and rewards them for their actions. We also want to coin to disappear, because it has been "picked up". Thanks to the simple API Leadwerks uses, this is extremely easy to do with the following code. Enter this in the MyCoin.lua script, run the game, and try to roll the ball over the coin to pick it up:
function Script:Start()
--Load a sound
self.sound = Sound:Load("Sound/coin.wav")
endfunction Script:Collision(entity, position, normal, speed)
--Make sure the sound exists and play it
if self.sound then
self.sound:Play()
end
--Hide the coin because it has been "picked up"
self.entity:Hide()
endfunction Script:UpdateWorld()
self.entity:Turn(0,Time:GetSpeed(),0,true)
endfunction Script:Release()
--If the sound was loaded, release it now and set the variable to nil
if self.sound then
self.sound:Release()
self.sound = nil
end
end
You can copy and paste the coin model to add more coins to collect. Now we're getting somewhere!:
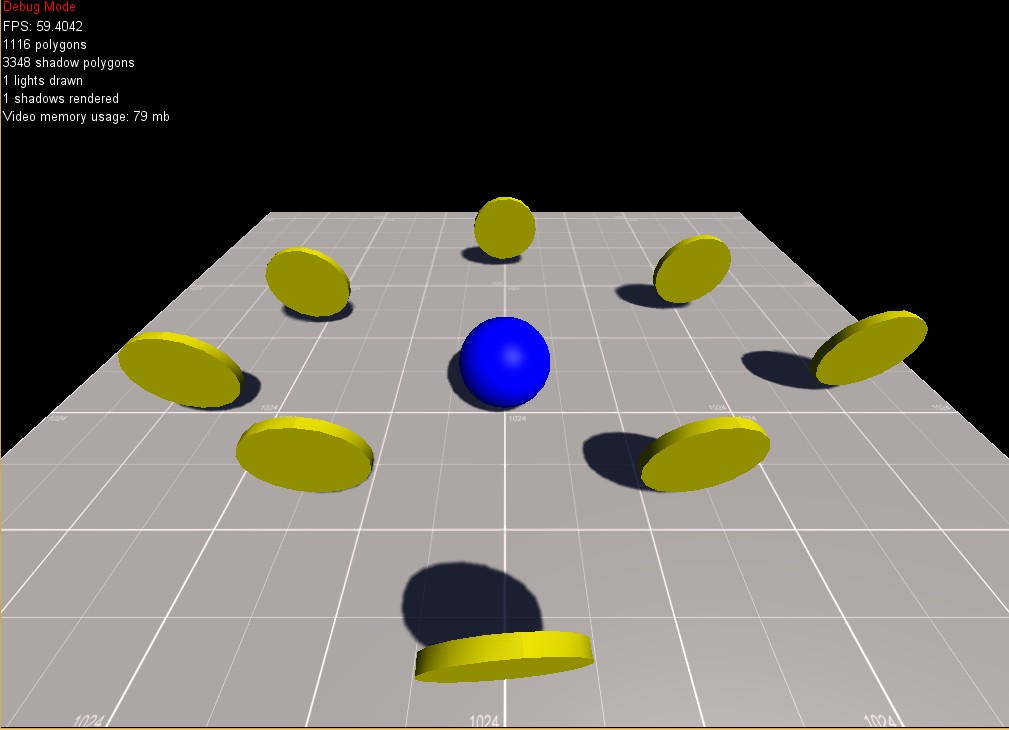